About a month ago I became interested in HTML5 audio. I already knew that the new HTML5 audio tags <audio> </audio> would add a default audio player to an HTML5 webpage. This is a good feature to have but I had questions about HTML5 audio…
The first question I had concerned the fact that the default audio player for me in my Firefox (v4 beta) browser the volume control would not work. I posted a question on Mozilla forum but have had no reply about this.
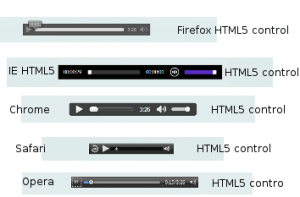
My next question was – how can I add my own custom controls and not use the default controls? I did some Google research and found the way to do this is with Javascript (or JQuery). I started to code my own HTML5 audio player with a view to making a player I could use here on my blog. As well as Javascript some styling is needed with CSS.
Well, after about 3 weeks of tinkering I finally finished my own basic HTML5 audio player that works here on my WordPress blog. I used the HTML5 code in a text widget and the player works and is located on the sidebar of the blog if you want to try it.
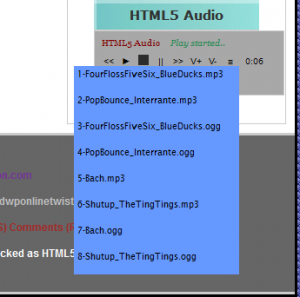
I would like to post a full explanation of my HTML5 audio player experience but this would expand into quite a big post!
However I should mention that in order to use HTML5 audio, both audio tags and a sound file source are needed. This is where things start to get trickier as of course different browsers support different audio file formats. Currently to work around this it is wise to provide multiple different audio source file formats (eg .mp3 .ogg .wav .mp4a etc) which is a sort of fallback effect:
<audio controls id="song">
<source src="mzm.ubzkrulq.aac.p.m4a">
<source src="letsgosurfing-thedrums.mp3">
<source src="letsgosurfing-thedrums.ogg">
</audio>
MP3 for Chrome, Internet Explorer, and Safari- Ogg Vorbis for Firefox and Opera & .ogg works in Firefox, Opera and Chrome while .mp3 is for Internet Explorer and Safari.
Given that a variety of audio sources need to be provided, it is good to have a good freeware audio converter program handy and Microsoft recommended FreeRip which is actually pretty good-see FreeRip
Example default controls HTML5 audio player
As seen from this code example the audio tag has an attribute ‘controls’ which provides the default browser HTML5 audio player control (with play/pause/vol/time functions).It can also be styled with CSS eg id=”song” etc.
The audio object has these types of members:Events/Methods/Properties
These are used inside audio tags as already seen
HTML5 property attributes (eg Controls/Preload/Autoplay/Loop):
- Controls gives the user agent (ie browser) default control with <audio controls> .It can also be coded as > controls=”controls” <
- These default controls include play/pause/progress/volume methods and css styling
- Preload – is a hint to the browser to load the audio file immediately the page is opened with <audio preload> – Gets or sets a hint to how much buffering is advisable for a media resource, even if autoplay is not specified.
- Autoplay – Gets or sets a value that indicates whether to start playing the media automatically.Autoplay means the audio player plays the audio file by default (use this carefully as most users do not want to hear sounds they have not chosen playing) with <audio autoplay>
- Loop – means to keep playing the audio file continually with <audio loop>
- src — Address of the resource (here audio file).Can be within opening audio tag or between audio tags
- crossorigin — How the element handles crossorigin requests
- mediagroup — Groups media elements together with an implicit MediaController
- loop — Whether to loop the media resource eg Gets or sets a flag that specifies whether playback should restart after it completes.
- muted — Whether to mute the media resource by default-Gets or sets a flag that indicates whether the audio (either audio or the audio track on video media) is muted.
- autobuffer-The autobuffer element is not supported by Internet Explorer 9. Use the preload element instead.
- buffered – Gets a collection of buffered time ranges.
- currentSrc – Gets the address or URL of the current media resource (video,audio) that is selected by IHTMLMediaElement.
- currentTime – Gets or sets the current playback position, in seconds.
- defaultPlaybackRate – Gets or sets the default playback rate when the user is not using fast foward or reverse for a video or audio resource.
- duration – Gets the duration, in seconds, of the current media resource, a NaN value if duration is not available, or Infinity if the media resource is streaming.
- ended – Gets information about whether the playback has ended or not.
- error – Gets an IHTMLMediaError object representing the current error state of the media element.
- initialTime – Gets the earliest possible position, in seconds, that the playback can begin.
- networkState – Gets the current network activity for the element.
- paused – Gets a flag that specifies whether playback is paused.
- playbackRate – Gets or sets the current speed for the media resource to play. This speed is expressed as a multiple of the normal speed of the media resource.
- played – Gets TimeRanges for the current media resource that has been played.
- seekable – Returns a TimeRanges object that represents the ranges of the current media resource that can be seeked.
- seeking – Gets a flag that indicates whether the the client is currently moving to a new playback position in the media resource.
- src – The address or URL of the a media resource (video, audio) that is to be considered.
- volume – Gets or sets the volume level for audio portions of the media element.
It is possible to use any combination of these attributes together eg with <audio controls preload autoplay>
Events
The audio object has these events.
canplaythrough – Occurs when playback to end is possible without requiring a stop for further buffering.
durationchange – Occurs when the duration attribute is updated.
emptied – Occurs when the media element is reset to its initial state.
ended – Occurs when the end of playback is reached.
loadeddata – Occurs when media data is loaded at the current playback position.
loadedmetadata – Occurs when the duration and dimensions of the media have been determined.
loadstart – Occurs when Internet Explorer begins looking for media data.
pause – Occurs when playback is paused.
play – Occurs when the play method is requested.
playing – Occurs when the audio or video has started playing.
progress – Occurs to indicate progress while downloading media data.
ratechange – Occurs when the playback rate is increased or decreased.
seeked – Occurs when the seek operation ends.
seeking – Occurs when the current playback position is moved.
stalled – Occurs when the download has stopped.
suspend – Occurs if the load operation has been intentionally halted.
timeupdate – Occurs to indicate the current playback position.
volumechange – Occurs when the volume is changed, or playback is muted or unmuted.
waiting – Occurs when playback stops because the next frame of a video resource is not available.
Methods
The audio object has these methods:
canPlayType – Returns a string that specifies whether the client can play a given media resource type.
load – Resets the IHTMLMediaElement and loads a new media resource.
pause – Pauses the current playback and sets paused to true.
play – Loads and starts playback of a media resource.
See msdn.microsoft.com audio object
Property attributes add functions automatically to HTML5 audio tags but instead these functions can be added by using HTML5 media code ie using the HTML5 audio methods and events (ie .play(); .duration(); etc methods & events ie onclick ondurationchange ontimeupdate etc)
For more on Media Events see
w3.org HTML Elements
Javascript example
HTML5 audio controlled via Javascript
Rather than using the default browser (user-agent) controls (ie play,volume etc)
it is possible to use custom controls styled with HTML & CSS & using Javascript for function (eg on button click to activate HTML5 audio code play(); etc).
Buttons or links can be used and styled as controls. Other elements can also be used eg CSS3 icons or special charsets
See www.whatwg.org
To do this simply do not use ‘controls’ attribute in <audio>tags and instead include the necessary javascript controls coding and with the html/html5 & css styling
<audio id="audioex" src="demo.ogg"></audio>
<script>
var audio = document.getElementById("audioex");
audio.addEventListener('MozAudioAvailable', audioAvailableFunction, false);
audio.addEventListener('loadedmetadata', loadedMetadataFunction, false);
audio.play();
</script>
<button onclick="getElementById('audioex').play();">Play</button>
<button onclick="getElementById('audioex').pause();getElementById('audioex').currentTime=0;">Stop</button>
Example HTML5 audio player with with Javascript control
Just two clickable buttons as controls..needs a bit more style!
PHEW!
If you want to make your own custom HTML5 audio player it is a good idea to try & understand these attributes and methods and be able to use them with Javascript and or JQuery and CSS. There are many resources available on the internet.
Two of my favourite custom HTML5 players are:
BBPlayer @ bbplayer
and Scott Andrews demo example @ Scott Andrews HTML5 audio
There is more to HTML5 Audio than first is apparent…ENJOY! 🙂
Leave a Reply